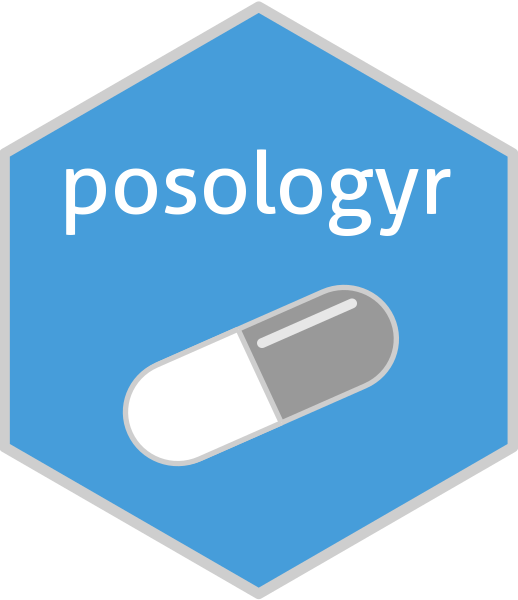
Estimate the optimal dose to achieve a target concentration at any given time
Source:R/dosing_optim.R
poso_dose_conc.Rd
Estimates the optimal dose to achieve a target concentration at any given time given a population pharmacokinetic model, a set of individual parameters, a selected point in time, and a target concentration.
Usage
poso_dose_conc(
dat = NULL,
prior_model = NULL,
tdm = FALSE,
time_c,
time_dose = NULL,
target_conc,
cmt_dose = 1,
endpoint = "Cc",
estim_method = "map",
nocb = FALSE,
p = NULL,
greater_than = TRUE,
starting_dose = 100,
interdose_interval = NULL,
add_dose = NULL,
duration = 0,
indiv_param = NULL
)
Arguments
- dat
Dataframe. An individual subject dataset following the structure of NONMEM/rxode2 event records.
- prior_model
A
posologyr
prior population pharmacokinetics model, a list of six objects.- tdm
A boolean. If
TRUE
: estimates the optimal dose for a selected target concentration at a selected point in time following the events fromdat
, and using Maximum A Posteriori estimation. Settingtdm
toTRUE
causes the following to occur:the arguments
estim_method
,p
,greater_than
,interdose_interval
,add_dose
,indiv_param
andstarting_time
are ignored.
- time_c
Numeric. Point in time for which the dose is to be optimized.
- time_dose
Numeric. Time when the dose is to be given.
- target_conc
Numeric. Target concentration.
- cmt_dose
Character or numeric. The compartment in which the dose is to be administered. Must match one of the compartments in the prior model. Defaults to 1.
- endpoint
Character. The endpoint of the prior model to be optimised for. The default is "Cc", which is the central concentration.
- estim_method
A character string. An estimation method to be used for the individual parameters. The default method "map" is the Maximum A Posteriori estimation, the method "prior" simulates from the prior population model, and "sir" uses the Sequential Importance Resampling algorithm to estimate the a posteriori distribution of the individual parameters. This argument is ignored if
indiv_param
is provided or iftdm
is set toTRUE
.- nocb
A boolean. for time-varying covariates: the next observation carried backward (nocb) interpolation style, similar to NONMEM. If
FALSE
, the last observation carried forward (locf) style will be used. Defaults toFALSE
.- p
Numeric. The proportion of the distribution of concentrations to consider for the optimization. Mandatory for
estim_method=sir
. This argument is ignored iftdm
is set toTRUE
.- greater_than
A boolean. If
TRUE
: targets a dose leading to a proportionp
of the concentrations to be greater thantarget_conc
. Respectively, lower ifFALSE
. This argument is ignored iftdm
is set toTRUE
.- starting_dose
Numeric. Starting dose for the optimization algorithm.
- interdose_interval
Numeric. Time for the interdose interval for multiple dose regimen. Must be provided when add_dose is used. This argument is ignored if
tdm
is set toTRUE
.- add_dose
Numeric. Additional doses administered at inter-dose interval after the first dose. Optional. This argument is ignored if
tdm
is set toTRUE
.- duration
Numeric. Duration of infusion, for zero-order administrations.
- indiv_param
Optional. A set of individual parameters : THETA, estimates of ETA, and covariates. This argument is ignored if
tdm
is set toTRUE
.
Value
A list containing the following components:
- dose
Numeric. An optimal dose for the selected target concentration.
- type_of_estimate
Character string. The type of estimate of the individual parameters. Either a point estimate, or a distribution.
- conc_estimate
A vector of numeric estimates of the conc. Either a single value (for a point estimate of ETA), or a distribution.
- indiv_param
A
data.frame
. The set of individual parameters used for the determination of the optimal dose : THETA, estimates of ETA, and covariates
Examples
rxode2::setRxThreads(2L) # limit the number of threads
# model
mod_run001 <- function() {
ini({
THETA_Cl <- 4.0
THETA_Vc <- 70.0
THETA_Ka <- 1.0
ETA_Cl ~ 0.2
ETA_Vc ~ 0.2
ETA_Ka ~ 0.2
prop.sd <- sqrt(0.05)
})
model({
TVCl <- THETA_Cl
TVVc <- THETA_Vc
TVKa <- THETA_Ka
Cl <- TVCl*exp(ETA_Cl)
Vc <- TVVc*exp(ETA_Vc)
Ka <- TVKa*exp(ETA_Ka)
K20 <- Cl/Vc
Cc <- centr/Vc
d/dt(depot) = -Ka*depot
d/dt(centr) = Ka*depot - K20*centr
Cc ~ prop(prop.sd)
})
}
# df_patient01: event table for Patient01, following a 30 minutes intravenous
# infusion
df_patient01 <- data.frame(ID=1,
TIME=c(0.0,1.0,14.0),
DV=c(NA,25.0,5.5),
AMT=c(2000,0,0),
EVID=c(1,0,0),
DUR=c(0.5,NA,NA))
# estimate the optimal dose to reach a concentration of 80 mg/l
# one hour after starting the 30-minutes infusion
poso_dose_conc(dat=df_patient01,prior_model=mod_run001,
time_c=1,duration=0.5,target_conc=80)
#>
#>
#> Error : cannot convert to rxUi object
#>
#>
#> Error : cannot convert to rxUi object
#> Error in priormod$ppk_model: object of type 'closure' is not subsettable