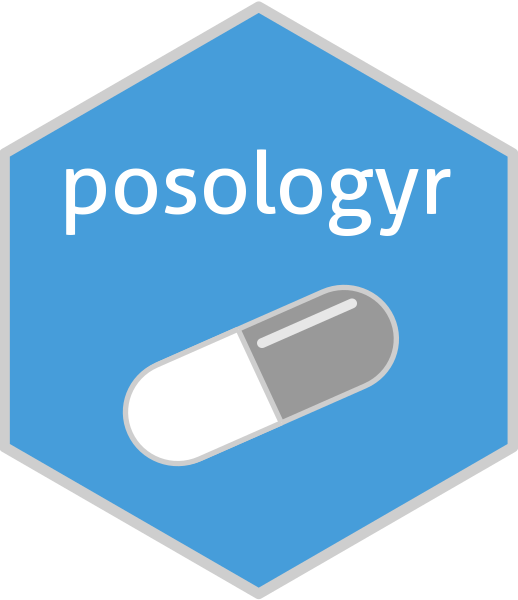
Estimate the time required to reach a target trough concentration (Cmin)
Source:R/dosing_optim.R
poso_time_cmin.Rd
Estimates the time required to reach a target trough concentration (Cmin) given a population pharmacokinetic model, a set of individual parameters, a dose, and a target Cmin.
Usage
poso_time_cmin(
dat = NULL,
prior_model = NULL,
tdm = FALSE,
target_cmin,
dose = NULL,
cmt_dose = 1,
endpoint = "Cc",
estim_method = "map",
nocb = FALSE,
p = NULL,
greater_than = TRUE,
from = 0.2,
last_time = 72,
add_dose = NULL,
interdose_interval = NULL,
duration = 0,
indiv_param = NULL
)
Arguments
- dat
Dataframe. An individual subject dataset following the structure of NONMEM/rxode2 event records.
- prior_model
A
posologyr
prior population pharmacokinetics model, a list of six objects.- tdm
A boolean. If
TRUE
: computes the predicted time to reach the target trough concentration (Cmin) following the last event fromdat
, and using Maximum A Posteriori estimation. Settingtdm
toTRUE
causes the following to occur:the simulation starts at the time of the last recorded dose (from the TDM data) plus
from
;the simulation stops at the time of the last recorded dose (from the TDM data) plus
last_time
;the arguments
dose
,duration
,estim_method
,p
,greater_than
,interdose_interval
,add_dose
,indiv_param
andstarting_time
are ignored.
- target_cmin
Numeric. Target trough concentration (Cmin).
- dose
Numeric. Dose administered. This argument is ignored if
tdm
is set toTRUE
.- cmt_dose
Character or numeric. The compartment in which the dose is to be administered. Must match one of the compartments in the prior model. Defaults to 1.
- endpoint
Character. The endpoint of the prior model to be optimised for. The default is "Cc", which is the central concentration.
- estim_method
A character string. An estimation method to be used for the individual parameters. The default method "map" is the Maximum A Posteriori estimation, the method "prior" simulates from the prior population model, and "sir" uses the Sequential Importance Resampling algorithm to estimate the a posteriori distribution of the individual parameters. This argument is ignored if
indiv_param
is provided, or iftdm
is set toTRUE
.- nocb
A boolean. For time-varying covariates: the next observation carried backward (nocb) interpolation style, similar to NONMEM. If
FALSE
, the last observation carried forward (locf) style will be used. Defaults toFALSE
.- p
Numeric. The proportion of the distribution of Cmin to consider for the estimation. Mandatory for
estim_method=sir
. This argument is ignored iftdm
is set toTRUE
.- greater_than
A boolean. If
TRUE
: targets a time leading to a proportionp
of the cmins to be greater thantarget_cmin
. Respectively, lower ifFALSE
. This argument is ignored iftdm
is set toTRUE
.- from
Numeric. Starting time for the simulation of the individual time-concentration profile. The default value is 0.2. When
tdm
is set toTRUE
the simulation starts at the time of the last recorded dose plusfrom
.- last_time
Numeric. Ending time for the simulation of the individual time-concentration profile. The default value is 72. When
tdm
is set toTRUE
the simulation stops at the time of the last recorded dose pluslast_time
.- add_dose
Numeric. Additional doses administered at inter-dose interval after the first dose. Optional. This argument is ignored if
tdm
is set toTRUE
.- interdose_interval
Numeric. Time for the inter-dose interval for multiple dose regimen. Must be provided when add_dose is used. This argument is ignored if
tdm
is set toTRUE
.- duration
Numeric. Duration of infusion, for zero-order administrations. This argument is ignored if
tdm
is set toTRUE
.- indiv_param
Optional. A set of individual parameters : THETA, estimates of ETA, and covariates.
Value
A list containing the following components:
- time
Numeric. Time needed to reach the selected Cmin.
- type_of_estimate
Character string. The type of estimate of the individual parameters. Either a point estimate, or a distribution.
- cmin_estimate
A vector of numeric estimates of the Cmin. Either a single value (for a point estimate of ETA), or a distribution.
- indiv_param
A
data.frame
. The set of individual parameters used for the determination of the time needed to reach a selected Cmin: THETA, estimates of ETA, and covariates
Examples
rxode2::setRxThreads(2L) # limit the number of threads
# model
mod_run001 <- function() {
ini({
THETA_Cl <- 4.0
THETA_Vc <- 70.0
THETA_Ka <- 1.0
ETA_Cl ~ 0.2
ETA_Vc ~ 0.2
ETA_Ka ~ 0.2
prop.sd <- sqrt(0.05)
})
model({
TVCl <- THETA_Cl
TVVc <- THETA_Vc
TVKa <- THETA_Ka
Cl <- TVCl*exp(ETA_Cl)
Vc <- TVVc*exp(ETA_Vc)
Ka <- TVKa*exp(ETA_Ka)
K20 <- Cl/Vc
Cc <- centr/Vc
d/dt(depot) = -Ka*depot
d/dt(centr) = Ka*depot - K20*centr
Cc ~ prop(prop.sd)
})
}
# df_patient01: event table for Patient01, following a 30 minutes intravenous
# infusion
df_patient01 <- data.frame(ID=1,
TIME=c(0.0,1.0,14.0),
DV=c(NA,25.0,5.5),
AMT=c(2000,0,0),
EVID=c(1,0,0),
DUR=c(0.5,NA,NA))
# predict the time needed to reach a concentration of 2.5 mg/l
# after the administration of a 2500 mg dose over a 30 minutes
# infusion
poso_time_cmin(dat=df_patient01,prior_model=mod_run001,
dose=2500,duration=0.5,from=0.5,target_cmin=2.5)
#>
#>
#> Error : cannot convert to rxUi object
#>
#>
#> Error : cannot convert to rxUi object
#> Error in priormod$ppk_model: object of type 'closure' is not subsettable